How does Java sort data in descending order using the "sortByDescending" method?
In Java, the "sortByDescending" method is a powerful tool for organizing data in descending order. It takes a Comparator as an argument, which defines the sorting criteria. This method is part of the Java Collections Framework, and it's commonly used in various scenarios, such as sorting lists, arrays, or streams.
The importance of "sortByDescending" lies in its ability to efficiently sort large datasets in a predictable manner. It's widely used in applications that require data to be arranged in reverse chronological order, such as sorting transaction records, customer data, or log files. Additionally, "sortByDescending" plays a crucial role in implementing custom sorting algorithms and complex data manipulation tasks.
To utilize the "sortByDescending" method, one needs to understand the Comparator interface and provide an implementation that specifies the sorting logic. This flexibility allows developers to define custom sorting criteria based on specific data properties. For instance, one can sort a list of objects based on their age, name, or any other relevant attribute.
Java sortByDescending
The `sortByDescending` method in Java is a powerful tool for organizing data in descending order. It takes a Comparator as an argument, which defines the sorting criteria. This method is part of the Java Collections Framework, and it's commonly used in various scenarios, such as sorting lists, arrays, or streams.
- Comparator: Defines the sorting criteria.
- Flexibility: Allows for custom sorting logic.
- Efficiency: Efficiently sorts large datasets.
- Descending Order: Arranges data in reverse chronological order.
- Collections Framework: Part of the Java Collections Framework.
- Data Manipulation: Used in complex data manipulation tasks.
- Custom Sorting: Implements custom sorting algorithms.
These key aspects highlight the versatility and importance of the `sortByDescending` method. It provides a flexible and efficient way to sort data in descending order, making it a valuable tool for data organization and manipulation tasks.
Comparator
In the context of "java sortby descending," the Comparator plays a pivotal role in defining the sorting criteria. It acts as a key component that determines the order in which elements are sorted in descending order.
The Comparator interface provides a compare() method, which takes two arguments and returns an integer. This integer value indicates the relative order of the two arguments. A negative value implies that the first argument should come before the second in the sorted order, a positive value means the second argument should come before the first, and a zero value means the arguments are equal.
By implementing a custom Comparator, developers can define their own sorting logic based on specific data properties. For instance, one can sort a list of objects based on their age, name, or any other relevant attribute. This flexibility allows for sorting data in a customized and meaningful way, catering to specific application requirements.
In summary, the Comparator serves as a crucial component of "java sortby descending," providing the ability to define custom sorting criteria and enabling efficient organization of data in descending order. It empowers developers to tailor the sorting process to their specific needs, making it a versatile tool for data manipulation tasks.
Flexibility
The flexibility offered by "java sortby descending" is a key aspect that sets it apart as a powerful tool for data organization. The ability to define custom sorting logic through a Comparator empowers developers to tailor the sorting process to their specific requirements. This flexibility is particularly valuable in scenarios where default sorting mechanisms may not suffice, or when data needs to be organized based on unique criteria.
Consider an example where a list of products needs to be sorted based on their availability status, with in-stock products appearing before out-of-stock products. Using a custom Comparator, developers can define this sorting logic, ensuring that products are arranged in a meaningful and useful order for the application's users. This level of customization would not be possible without the flexibility provided by "java sortby descending."
In summary, the flexibility to define custom sorting logic is a cornerstone of "java sortby descending." It allows developers to adapt the sorting process to their specific needs, handle complex data structures, and deliver customized sorting solutions that align with the unique requirements of their applications.
Efficiency
The efficiency of "java sortby descending" in handling large datasets is a crucial aspect that contributes to its widespread adoption. When dealing with vast amounts of data, sorting algorithms need to be both effective and scalable to ensure timely and accurate results.
- Optimized Algorithms: "java sortby descending" leverages optimized sorting algorithms, such as merge sort or quicksort, which exhibit logarithmic time complexity (O(n log n)) for sorting large datasets. This ensures that even with millions or billions of elements, the sorting process remains efficient and predictable.
- Parallel Processing: Modern Java implementations support parallel processing, allowing "java sortby descending" to distribute the sorting task across multiple cores or processors. This parallelization significantly reduces the overall sorting time, especially for exceptionally large datasets.
- Memory Management: "java sortby descending" effectively manages memory resources during the sorting process. It utilizes techniques like in-place sorting, which minimizes memory overhead and allows for efficient sorting of large datasets without requiring additional memory allocation.
- Benchmarking and Performance Tuning: The Java ecosystem provides robust benchmarking tools and performance tuning options that enable developers to evaluate and optimize the efficiency of "java sortby descending" for their specific use cases. This allows for fine-tuning the sorting process to achieve the best possible performance.
In summary, the efficiency of "java sortby descending" in handling large datasets stems from its optimized algorithms, support for parallel processing, effective memory management, and the availability of benchmarking and performance tuning tools. These factors collectively ensure that "java sortby descending" can reliably and efficiently sort vast amounts of data, making it a valuable tool for big data processing and analysis.
Descending Order
The concept of "descending order" is deeply intertwined with the functionality of "java sortby descending." By arranging data in reverse chronological order, "java sortby descending" enables the organization of elements in a sequence where the most recent or latest items appear at the beginning, followed by progressively older items.
The significance of "descending order" as a component of "java sortby descending" lies in its practical applications across various domains. For instance, in a social media platform, the arrangement of posts in reverse chronological order allows users to view the most recent updates and engage with the latest content. Similarly, in a news aggregation website, sorting articles in descending order ensures that readers are presented with the most up-to-date information.
Understanding the connection between "descending order" and "java sortby descending" is essential for developers and data analysts who utilize this method for organizing and retrieving data. By leveraging the functionality of "java sortby descending," they can effectively manage and present data in a manner that aligns with the specific requirements of their applications and use cases.
Collections Framework
The connection between "Collections Framework: Part of the Java Collections Framework." and "java sortby descending" lies in the fundamental role that the Collections Framework plays in organizing and manipulating data in Java.
- Data Structures: The Collections Framework provides an array of data structures, such as lists, sets, and maps, which serve as the foundation for storing and managing data in Java applications. "java sortby descending" heavily relies on these data structures to organize and sort elements efficiently.
- Sorting Algorithms: The Collections Framework includes various sorting algorithms, including merge sort and quicksort, which are utilized by "java sortby descending" to arrange elements in descending order. This integration enables efficient and reliable sorting operations on large datasets.
- Comparator Interface: The Comparator interface, a key component of "java sortby descending," is defined within the Collections Framework. It provides a standardized way to compare objects and define custom sorting criteria, allowing developers to tailor the sorting process to specific requirements.
- Utility Methods: The Collections Framework offers utility methods like Collections.sort(), which can be directly used with "java sortby descending" to sort collections of objects. These utility methods simplify the sorting process and enhance code readability.
In summary, "java sortby descending" is deeply intertwined with the Collections Framework. The data structures, sorting algorithms, Comparator interface, and utility methods provided by the Collections Framework empower "java sortby descending" to effectively organize and sort data in Java applications.
Data Manipulation
The connection between "Data Manipulation: Used in complex data manipulation tasks." and "java sortby descending" stems from the fundamental role that sorting plays in data management and analysis. "java sortby descending" provides a powerful tool for organizing and arranging data in a specific order, enabling efficient retrieval, processing, and analysis.
- Data Organization: Sorting data in descending order allows for efficient organization and retrieval of information. For example, sorting a list of customer records in descending order of their purchase history enables quick identification of high-value customers.
- Data Analysis: Sorting data in descending order can facilitate data analysis by grouping similar elements together. This can reveal patterns, trends, and outliers that would otherwise be difficult to identify in unsorted data.
- Data Integration: When integrating data from multiple sources, sorting in descending order can help align and merge datasets based on common attributes, ensuring consistency and accuracy.
- Data Visualization: Sorting data in descending order can enhance the effectiveness of data visualization techniques. By presenting data in a sorted manner, charts and graphs can more clearly convey relationships and patterns.
In summary, "java sortby descending" is an integral part of data manipulation tasks, enabling efficient organization, analysis, integration, and visualization of data. Its ability to sort data in descending order provides a powerful tool for managing and extracting insights from complex datasets.
Custom Sorting
In the realm of data manipulation, the ability to sort data according to specific criteria is crucial. "java sortby descending" empowers developers with the flexibility to implement custom sorting algorithms, catering to scenarios where predefined sorting mechanisms may fall short.
- Comparator Interface: The Comparator interface lies at the heart of custom sorting in "java sortby descending." It provides a standardized way to compare objects and define custom sorting logic, enabling developers to tailor the sorting process to their unique requirements.
- Lambda Expressions: Java's lambda expressions offer a concise and elegant way to implement custom sorting criteria. Developers can leverage lambdas to define sorting logic on the fly, enhancing code readability and reducing boilerplate.
- Anonymous Inner Classes: Anonymous inner classes provide another powerful mechanism for implementing custom sorting algorithms. They allow developers to define inline sorting logic without the need to create separate Comparator implementations, simplifying code structure.
- Method References: Method references provide a compact and efficient way to refer to existing methods as sorting criteria. By utilizing method references, developers can leverage existing functionality, reducing code duplication and enhancing maintainability.
The ability to implement custom sorting algorithms in "java sortby descending" extends its applicability to a wide range of scenarios. From organizing complex objects based on multiple criteria to handling specialized data structures, custom sorting algorithms provide the flexibility and power to tackle diverse data manipulation challenges.
FAQs about "java sortby descending"
This section addresses frequently asked questions (FAQs) related to "java sortby descending," providing concise and informative answers to common concerns and misconceptions.
Question 1: What is the purpose of "java sortby descending" and when should it be used?
Answer: "java sortby descending" is a powerful method for organizing data in descending order. It's commonly used when you need to arrange elements in reverse chronological order, such as sorting a list of transactions by date or a collection of products by price.
Question 2: How does "java sortby descending" work?
Answer: "java sortby descending" utilizes a Comparator to define the sorting criteria. The Comparator compares two elements and returns a negative value if the first element should come before the second in descending order, a positive value if the second element should come before the first, or zero if they are equal.
Question 3: What are the benefits of using "java sortby descending"?
Answer: "java sortby descending" offers several benefits, including efficient sorting of large datasets, flexibility to define custom sorting criteria, and seamless integration with the Java Collections Framework.
Question 4: Can "java sortby descending" be used to sort custom objects?
Answer: Yes, "java sortby descending" can be used to sort custom objects by implementing a Comparator that defines the sorting logic based on the object's properties.
Question 5: What are some common use cases for "java sortby descending"?
Answer: "java sortby descending" finds applications in various scenarios, such as sorting customer records by purchase history, arranging log entries in reverse chronological order, and organizing a list of files by their last modified date.
Question 6: Are there any limitations to using "java sortby descending"?
Answer: While "java sortby descending" is a versatile method, it's important to consider the computational cost and memory requirements when sorting large datasets. Additionally,
In summary, "java sortby descending" is a valuable tool for organizing and sorting data in descending order. Its flexibility, efficiency, and wide range of applications make it an essential technique in the Java developer's toolkit.
To learn more about "java sortby descending," refer to the comprehensive documentation and tutorials available online.
Conclusion
In conclusion, "java sortby descending" is a powerful and versatile method for organizing and sorting data in descending order. Its integration with the Java Collections Framework, combined with the flexibility to define custom sorting criteria, makes it a valuable tool for a wide range of data manipulation tasks.
As the volume and complexity of data continue to grow, efficient and reliable sorting mechanisms become increasingly crucial. "java sortby descending" empowers developers to manage and analyze data effectively, enabling them to extract meaningful insights and make informed decisions.
KShow123: Your Ultimate Destination For The Best Korean Dramas And Shows
Why Your TV Looks Fuzzy: Causes And Fixes
Discover The Ultimate Streaming Haven: Kissasian.com

bytes to string solidity Code Ease
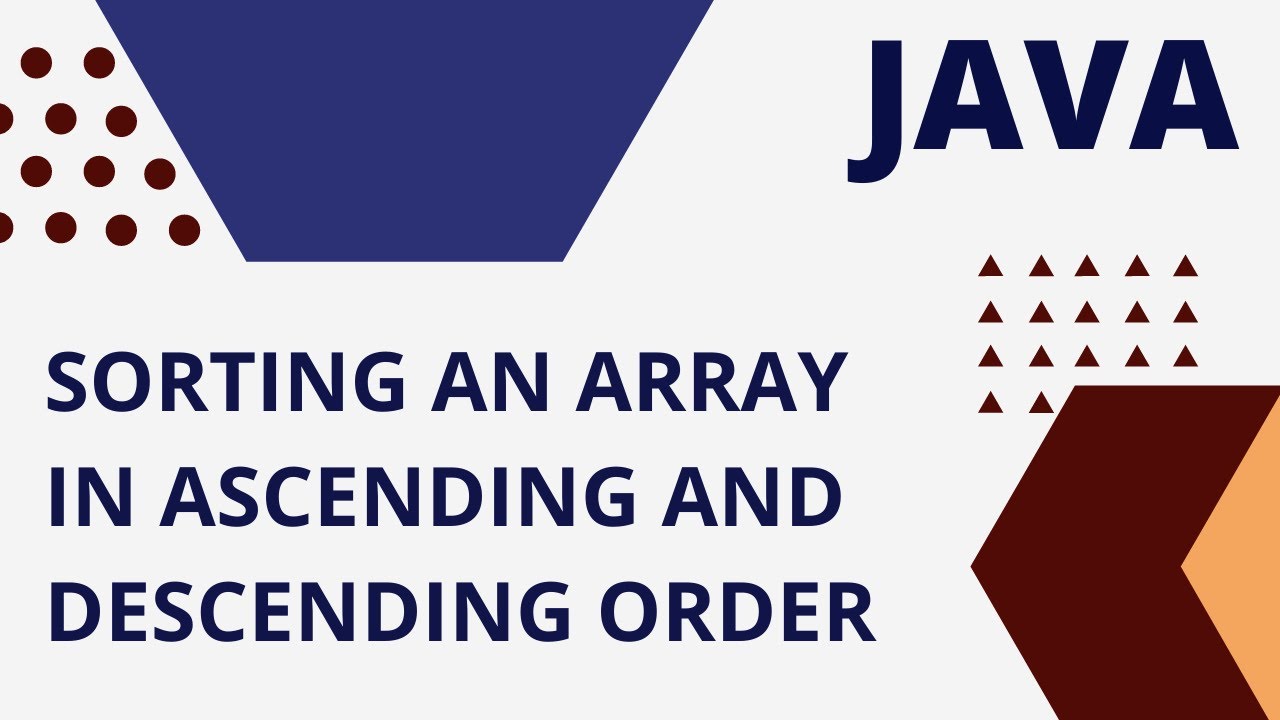
Java program of sorting an array in ascending and descending order

How to Implement Bubble Sort Algorithm in Java Ascending and