What is Oracle SQL IF AND?
Oracle SQL IF AND is a conditional statement used to control the flow of execution in a PL/SQL block. It evaluates a specified condition and executes a set of statements if the condition is met.
The syntax of the IF AND statement is as follows:
IF condition THEN statementsEND IF;
For example, the following IF AND statement checks if the value of the variable x
is greater than 10, and if so, it prints the message "x is greater than 10":
IF x > 10 THEN DBMS_OUTPUT.PUT_LINE('x is greater than 10');END IF;
The IF AND statement can be used to control the flow of execution in a variety of ways. It can be used to execute different sets of statements depending on the value of a variable, to skip over sections of code, or to handle errors.
The IF AND statement is a powerful tool that can be used to improve the control flow of your PL/SQL code. It is important to understand how the IF AND statement works in order to use it effectively.
Oracle SQL IF AND
Oracle SQL IF AND is a conditional statement used to control the flow of execution in a PL/SQL block. It evaluates a specified condition and executes a set of statements if the condition is met.
- Syntax: IF condition THEN statements END IF;
- Purpose: Controls the flow of execution in a PL/SQL block
- Condition: Evaluates a specified condition
- Statements: Executes a set of statements if the condition is met
- Usage: Can be used to execute different sets of statements depending on the value of a variable, to skip over sections of code, or to handle errors
The Oracle SQL IF AND statement is a powerful tool that can be used to improve the control flow of your PL/SQL code. It is important to understand how the IF AND statement works in order to use it effectively.
Syntax
The syntax "IF condition THEN statements END IF;" is the foundation of the Oracle SQL IF AND statement. It defines the structure and components of the statement, enabling it to control the flow of execution in a PL/SQL block.
The IF condition evaluates a specified condition, such as whether a variable is greater than a certain value or whether a particular condition is met. If the condition is met, the statements following the THEN keyword are executed. Otherwise, the statements are skipped, and execution continues with the statement following the END IF keyword.
For example, the following IF AND statement checks if the value of the variable x
is greater than 10. If it is, the statement "DBMS_OUTPUT.PUT_LINE('x is greater than 10')
" is executed, printing the message "x is greater than 10" to the console.
IF x > 10 THEN DBMS_OUTPUT.PUT_LINE('x is greater than 10');END IF;
The Oracle SQL IF AND statement is a powerful tool that can be used to control the flow of execution in a variety of ways. It can be used to execute different sets of statements depending on the value of a variable, to skip over sections of code, or to handle errors.
Understanding the syntax of the Oracle SQL IF AND statement is essential for using it effectively in your PL/SQL code.
Purpose
The Oracle SQL IF AND statement is a conditional statement used to control the flow of execution in a PL/SQL block. This means that it allows you to specify different paths of execution based on whether a specified condition is met or not.
- Conditional Execution: The IF AND statement allows you to execute specific statements only if a certain condition is met. This enables you to create more complex and dynamic PL/SQL code that can adapt to different scenarios.
- Error Handling: You can use the IF AND statement to handle errors and exceptions in your PL/SQL code. By checking for specific error conditions, you can gracefully handle errors and provide meaningful error messages to users.
- Code Reusability: The IF AND statement can help you improve the reusability of your PL/SQL code. By encapsulating conditional logic in separate IF AND statements, you can easily reuse that logic in different parts of your code.
Overall, the IF AND statement is a powerful tool that gives you control over the flow of execution in your PL/SQL code. By understanding how to use the IF AND statement effectively, you can write more robust, efficient, and maintainable PL/SQL code.
Condition
The condition in an Oracle SQL IF AND statement is a logical expression that evaluates to either TRUE or FALSE. If the condition evaluates to TRUE, the statements following the THEN keyword are executed. Otherwise, the statements are skipped, and execution continues with the statement following the END IF keyword.
- Syntax: The syntax for the condition in an IF AND statement is the same as for any other logical expression in Oracle SQL. It can include any combination of operands, operators, and parentheses.
- Examples: Here are a few examples of valid conditions in IF AND statements:
- x > 10
- name = 'John Doe'
- NOT (salary IS NULL)
- Implications: The condition in an IF AND statement is crucial because it determines whether the statements following the THEN keyword are executed. By carefully crafting the condition, you can control the flow of execution in your PL/SQL code and ensure that only the necessary statements are executed.
Overall, the condition in an Oracle SQL IF AND statement is a powerful tool that allows you to control the flow of execution in your PL/SQL code. By understanding how to write and evaluate conditions, you can write more efficient and effective PL/SQL code.
Statements
In Oracle SQL IF AND statements, the statements following the THEN keyword are executed only if the condition evaluates to TRUE. This allows you to control the flow of execution in your PL/SQL code and execute different sets of statements depending on the value of a variable, the state of the database, or any other condition.
For example, the following IF AND statement checks if the value of the variable x
is greater than 10. If it is, the statement "DBMS_OUTPUT.PUT_LINE('x is greater than 10')
" is executed, printing the message "x is greater than 10" to the console.
IF x > 10 THEN DBMS_OUTPUT.PUT_LINE('x is greater than 10');END IF;
The ability to execute a set of statements only if a condition is met is essential for writing complex and dynamic PL/SQL code. It allows you to handle different scenarios, perform error handling, and improve the overall flow of your code.
Understanding the connection between "Statements: Executes a set of statements if the condition is met" and "oracle sql if and" is crucial for writing effective PL/SQL code. By mastering the use of IF AND statements, you can control the flow of execution in your code and write more robust and efficient programs.
Usage
The "Usage: Can be used to execute different sets of statements depending on the value of a variable, to skip over sections of code, or to handle errors" is a fundamental aspect of the Oracle SQL IF AND statement, empowering it to control the flow of execution in PL/SQL code.
By evaluating a specified condition, the IF AND statement determines whether to execute the statements following the THEN keyword. This capability enables developers to execute different sets of statements based on the value of a variable, skip over sections of code that are not applicable, or handle errors gracefully.
For example, consider a scenario where you want to process customer orders based on their order status. Using an IF AND statement, you can define different sets of statements to handle each order status, such as "Processing," "Shipped," or "Delivered." This allows you to execute only the necessary statements for each order, improving the efficiency and accuracy of your code.
Furthermore, the IF AND statement is crucial for handling errors and exceptions in PL/SQL code. By checking for specific error conditions, you can gracefully handle errors, provide meaningful error messages to users, and prevent unexpected program termination.
In summary, the "Usage: Can be used to execute different sets of statements depending on the value of a variable, to skip over sections of code, or to handle errors" is a critical component of the Oracle SQL IF AND statement, enabling developers to write robust, dynamic, and error-resilient PL/SQL code.
FAQs on Oracle SQL IF AND
This section addresses frequently asked questions about the Oracle SQL IF AND statement to provide a comprehensive understanding of its usage and significance.
Question 1: What is the purpose of the Oracle SQL IF AND statement?
The Oracle SQL IF AND statement is a conditional statement used to control the flow of execution in a PL/SQL block. It evaluates a specified condition and executes a set of statements if the condition is met.
Question 2: What is the syntax of the Oracle SQL IF AND statement?
The syntax of the Oracle SQL IF AND statement is as follows:
IF condition THENstatementsEND IF;
Question 3: How can the Oracle SQL IF AND statement be used to handle errors?
The Oracle SQL IF AND statement can be used to handle errors by checking for specific error conditions. If an error condition is met, the IF AND statement can execute a set of statements to handle the error gracefully.
Question 4: What is the difference between the Oracle SQL IF AND statement and the Oracle SQL IF statement?
The Oracle SQL IF AND statement evaluates multiple conditions using the AND operator, while the Oracle SQL IF statement evaluates a single condition. The IF AND statement is used when you need to check multiple conditions before executing a set of statements.
Question 5: Can the Oracle SQL IF AND statement be used to skip over sections of code?
Yes, the Oracle SQL IF AND statement can be used to skip over sections of code by evaluating a condition and only executing the statements following the THEN keyword if the condition is met.
Question 6: How can the Oracle SQL IF AND statement be used to improve the readability and maintainability of code?
The Oracle SQL IF AND statement can be used to improve the readability and maintainability of code by organizing code into logical blocks and making it easier to understand the flow of execution.
These FAQs provide a solid foundation for understanding the Oracle SQL IF AND statement and its various applications in PL/SQL development.
Moving forward, let's explore the benefits and practical applications of the Oracle SQL IF AND statement in greater detail.
Conclusion
Throughout this exploration of "Oracle SQL IF AND", we have delved into its purpose, syntax, usage, and significance in PL/SQL development. The Oracle SQL IF AND statement has proven to be a powerful tool for controlling the flow of execution, handling errors, and improving code readability and maintainability.
As we move forward, the Oracle SQL IF AND statement will continue to be an essential tool for PL/SQL developers. Its ability to evaluate conditions and execute specific sets of statements makes it a versatile and indispensable component of PL/SQL programming. By mastering the use of the Oracle SQL IF AND statement, developers can write robust, efficient, and maintainable PL/SQL code that meets the demands of modern database applications.
Ultimate Guide To Wmoov: Empowering Your Digital Marketing Strategy
How Long Do Korean Side Dishes Keep In The Fridge: A Guide To Freshness
A Comprehensive Guide To Diaphysis, Metaphysis, And Epiphysis

Oracle PL/SQL developer data generator Stack Overflow
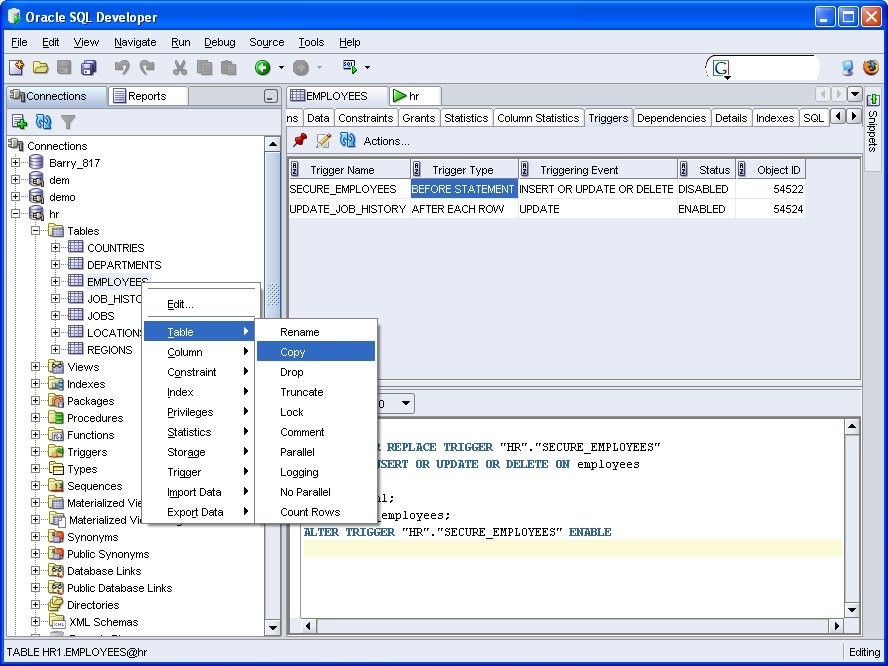
Oracle SQL Developer Release 3.2 Here Free by Oracle Your Post My Blog

Скачать бесплатно Oracle SQL Developer для компьютера под Windows