Need to merge two number columns into one in SQL? You're not alone. This is a common task that can be accomplished in a few different ways.
One way to merge two number columns into one is to use the CONCAT()
function. This function takes two strings as arguments and returns a new string that is the concatenation of the two arguments. For example, the following query would merge the first_name
and last_name
columns into a new column called full_name
:
SELECT CONCAT(first_name, ' ', last_name) AS full_nameFROM table_name;
Another way to merge two number columns into one is to use the +
operator. This operator can be used to add two numbers together. For example, the following query would add the quantity
and price
columns to create a new column called total
:
SELECT quantity + price AS totalFROM table_name;
Which method you use to merge two number columns into one will depend on your specific needs. If you need to create a new string, then the CONCAT()
function is a good option. If you need to add two numbers together, then the +
operator is a good option.
No matter which method you choose, merging two number columns into one is a simple task that can be accomplished in a few easy steps.
Two Number Columns to One SQL
Merging two number columns into one is a common task in SQL. There are several ways to do this, depending on your specific needs. Here are six key aspects to consider:
- Data types: The data types of the two columns must be compatible. For example, you cannot concatenate a text column and a number column.
- Column names: The new column will have a new name. You can specify the new column name using the
AS
keyword. - Order of operations: The order of operations is important when using multiple operators. For example, the
+
operator has a lower precedence than the*
operator. - NULL values: NULL values can cause problems when merging columns. You can use the
COALESCE()
function to handle NULL values. - Performance: The performance of your query can be affected by the method you use to merge columns. The
CONCAT()
function is generally faster than the+
operator. - Readability: Your code should be readable and easy to understand. Use descriptive column names and avoid complex expressions.
By considering these six aspects, you can merge two number columns into one in SQL efficiently and effectively.
Data types
When merging two number columns into one in SQL, it is important to ensure that the data types of the two columns are compatible. This means that the columns must have the same data type, such as integer, float, or decimal. If the columns have different data types, the query will fail.
- Example: The following query will fail because the
first_name
column is a text column and theage
column is a number column:SELECT CONCAT(first_name, age) AS full_nameFROM table_name;
- Solution: To fix this query, you can convert the
age
column to a text column using theCAST()
function:SELECT CONCAT(first_name, CAST(age AS TEXT)) AS full_nameFROM table_name;
By ensuring that the data types of the two columns are compatible, you can avoid errors and ensure that your query returns the correct results.
Column names
When merging two number columns into one in SQL, it is important to specify the name of the new column. This is done using the AS
keyword. The AS
keyword is used to assign an alias to a column. The alias can be any valid SQL identifier.
For example, the following query merges two number columns, quantity
and price
, into a new column called total
:
SELECT quantity + price AS total FROM table_name;
In this example, the AS
keyword is used to assign the alias total
to the new column. The alias total
can then be used to refer to the new column in the rest of the query.
Specifying the name of the new column is important for several reasons. First, it makes the query more readable and easier to understand. Second, it allows you to use the new column in other parts of the query, such as in the WHERE
clause or the ORDER BY
clause.
By specifying the name of the new column, you can ensure that your query is clear, concise, and easy to understand.
Order of operations
When merging two number columns into one in SQL, it is important to be aware of the order of operations. The order of operations determines the order in which the operations in a query are performed. This is important because it can affect the results of the query.
For example, the following query merges two number columns, quantity
and price
, into a new column called total
:
SELECT quantity + price discount AS total FROM table_name;
In this example, the +
operator has a lower precedence than the operator. This means that the operator will be performed first, and the +
operator will be performed second. As a result, the discount
will be applied to the price
before the quantity
is added.
If the order of operations were reversed, the results of the query would be different. For example, the following query would add the quantity
and price
together before applying the discount
:
SELECT quantity * price + discount AS total FROM table_name;
By understanding the order of operations, you can ensure that your queries return the correct results.
Here is a summary of the order of operations in SQL:
- Parentheses
- Exponents
- Multiplication and division
- Addition and subtraction
By following the order of operations, you can avoid errors and ensure that your queries return the correct results.
NULL values
When merging two number columns into one in SQL, it is important to consider the possibility of NULL values. NULL values can occur when a value is missing or unknown. If one of the columns contains NULL values, the query may return an error.
To handle NULL values, you can use the COALESCE()
function. The COALESCE()
function takes two or more arguments. If the first argument is NULL, the COALESCE()
function returns the second argument. If the second argument is NULL, the COALESCE()
function returns the third argument, and so on.
For example, the following query merges two number columns, quantity
and price
, into a new column called total
. The COALESCE()
function is used to handle NULL values in the quantity
column:
SELECT COALESCE(quantity, 0) + price AS total FROM table_name;
In this example, if the quantity
column contains a NULL value, the COALESCE()
function will return 0. This will prevent the query from returning an error.
The COALESCE()
function is a powerful tool for handling NULL values. By using the COALESCE()
function, you can ensure that your queries return the correct results, even when there are NULL values in the data.
Performance
When merging two number columns into one in SQL, it is important to consider the performance of the query. The performance of the query can be affected by several factors, including the method you use to merge the columns.
- Method: There are two main methods for merging two number columns into one: the
CONCAT()
function and the+
operator. TheCONCAT()
function is generally faster than the+
operator, especially when merging large amounts of data. - Data types: The data types of the two columns can also affect the performance of the query. For example, merging two integer columns will be faster than merging two text columns.
- Number of columns: The number of columns you are merging can also affect the performance of the query. Merging a large number of columns will be slower than merging a small number of columns.
By considering these factors, you can choose the best method for merging two number columns into one in SQL. In most cases, the CONCAT()
function is the best choice, especially when merging large amounts of data.
Readability
When merging two number columns into one in SQL, it is important to consider the readability of your code. Readable code is code that is easy to understand and maintain. This is important for several reasons.
- Code maintenance: Readable code is easier to maintain. This is because it is easier to understand what the code is doing and how it is doing it. This makes it easier to make changes to the code in the future, such as fixing bugs or adding new features.
- Collaboration: Readable code is easier to collaborate on. This is because it is easier for other developers to understand what the code is doing and how it is doing it. This makes it easier to work together on projects and to share code.
- Debugging: Readable code is easier to debug. This is because it is easier to identify and fix errors in the code. This can save time and frustration when trying to fix a problem.
There are several things you can do to improve the readability of your code. One important thing is to use descriptive column names. This will make it easier to understand what the data in each column represents. For example, instead of using the column name col1
, you could use the column name customer_name
.
Another important thing is to avoid complex expressions. Complex expressions can be difficult to understand and maintain. Instead, try to break down complex expressions into smaller, more manageable pieces. This will make your code easier to read and understand.
By following these tips, you can improve the readability of your code. This will make your code easier to maintain, collaborate on, and debug.
Two Number Columns to One SQL FAQs
This section answers some of the most frequently asked questions about merging two number columns into one in SQL.
Question 1: What is the difference between the CONCAT()
function and the +
operator?
Answer: The CONCAT()
function concatenates two strings together, while the +
operator adds two numbers together. When merging two number columns into one, the CONCAT()
function is generally faster than the +
operator.
Question 2: How do I handle NULL values when merging two number columns?
Answer: You can use the COALESCE()
function to handle NULL values. The COALESCE()
function takes two or more arguments. If the first argument is NULL, the COALESCE()
function returns the second argument. If the second argument is NULL, the COALESCE()
function returns the third argument, and so on.
Question 3: How do I improve the performance of my query when merging two number columns?
Answer: There are several things you can do to improve the performance of your query when merging two number columns. One important thing is to use the CONCAT()
function instead of the +
operator. Another important thing is to avoid using complex expressions.
Question 4: How do I make my code more readable when merging two number columns?
Answer: There are several things you can do to make your code more readable when merging two number columns. One important thing is to use descriptive column names. Another important thing is to avoid complex expressions.
Question 5: What are some common mistakes to avoid when merging two number columns?
Answer: Some common mistakes to avoid when merging two number columns include using the wrong data types, not handling NULL values properly, and using inefficient methods.
Question 6: Where can I learn more about merging two number columns in SQL?
Answer: There are many resources available online to learn more about merging two number columns in SQL. Some good resources include the official SQL documentation, tutorials, and online forums.
By following these tips, you can merge two number columns into one in SQL efficiently and effectively.
Transition to the next article section:
Now that you have learned about merging two number columns into one in SQL, you can move on to the next section, which will discuss more advanced topics.
Conclusion
In this article, we have explored the topic of merging two number columns into one in SQL. We have discussed the different methods that can be used to merge columns, as well as the advantages and disadvantages of each method. We have also provided some tips for improving the performance and readability of your code.
Merging two number columns into one is a common task in SQL. By understanding the different methods that are available, you can choose the best method for your specific needs. By following the tips in this article, you can also improve the performance and readability of your code.
A Comprehensive Examination Of Ordinal Variables: Definition, Analysis, And Applications
The Case In Point: A Practical Example
The Perils Of Rationality: Unmasking The Irrationality Of McDonaldization
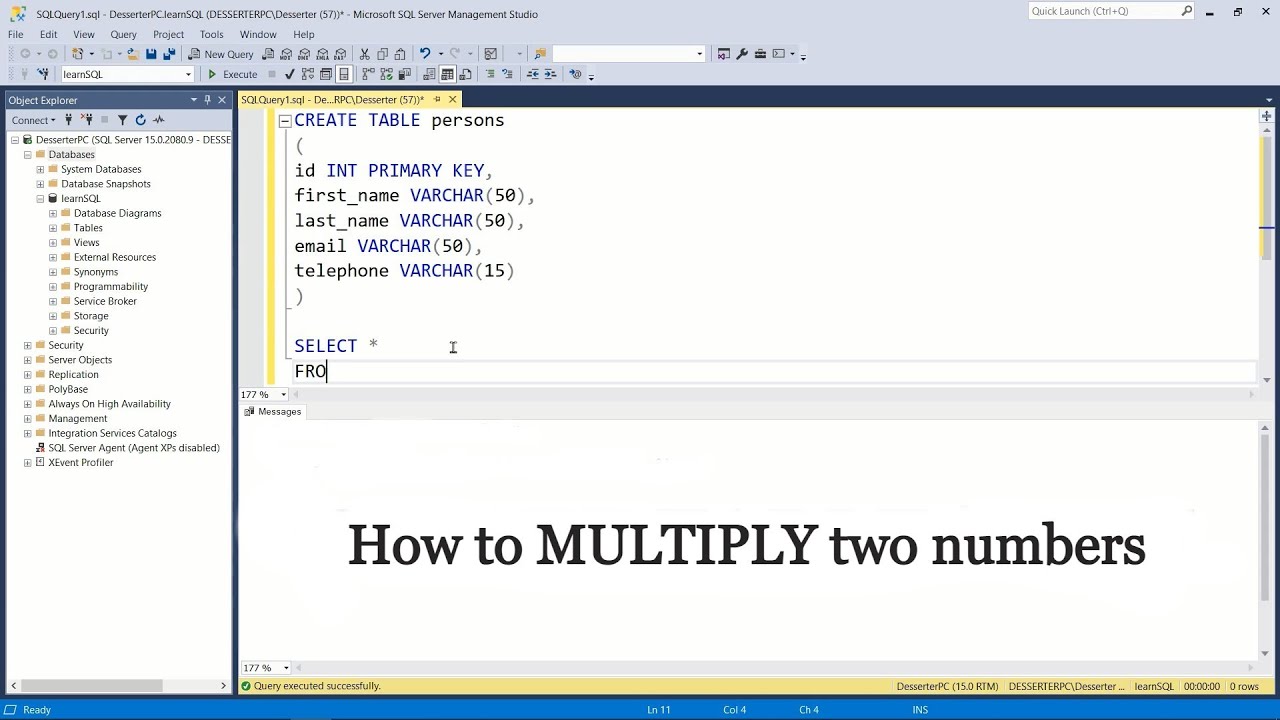
How to MULTIPLY TWO NUMBERS in SQL YouTube
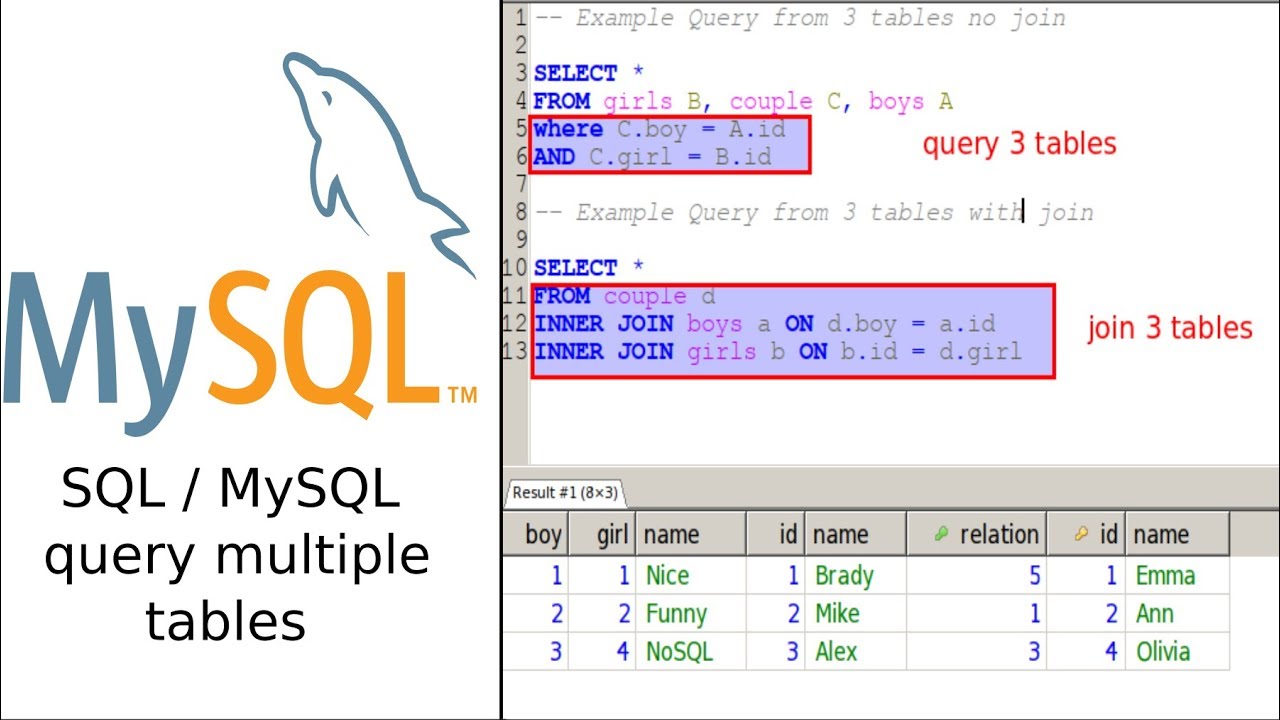
Php Mysql Select From Multiple Tables? Top 2 Best Answers

Printable Columns And Rows Printable Word Searches